迭代器模式(Iterator Pattern)是一种行为型设计模式,它提供了一种顺序访问聚合对象中各个元素的方法,而不需要暴露聚合对象的内部结构。
迭代器模式中通常包含以下角色:文章源自新逸网络-https://www.xinac.net/9364.html
1. 抽象迭代器(Iterator):定义了访问和遍历聚合对象的接口,包含了一些基本的方法,例如 `hasNext()`、`next()` 等。文章源自新逸网络-https://www.xinac.net/9364.html
2. 具体迭代器(Concrete Iterator):实现了抽象迭代器接口,用于具体实现访问和遍历聚合对象的操作。文章源自新逸网络-https://www.xinac.net/9364.html
3. 抽象聚合类(Aggregate):定义了聚合对象的接口,包含了一些基本的方法,例如 `add()`、`remove()`、`iterator()` 等。文章源自新逸网络-https://www.xinac.net/9364.html
4. 具体聚合类(Concrete Aggregate):实现了抽象聚合类接口,用于具体实现聚合对象的操作。文章源自新逸网络-https://www.xinac.net/9364.html
下面是一个简单的 Java 实现迭代器模式的示例:文章源自新逸网络-https://www.xinac.net/9364.html
// 抽象迭代器 interface Iterator { boolean hasNext(); Object next(); } // 具体迭代器 class ConcreteIterator implements Iterator { private String[] names; private int index = 0; public ConcreteIterator(String[] names) { this.names = names; } public boolean hasNext() { return index < names.length; } public Object next() { if (hasNext()) { return names[index++]; } return null; } } // 抽象聚合类 interface Aggregate { void add(Object obj); void remove(Object obj); Iterator iterator(); } // 具体聚合类 class ConcreteAggregate implements Aggregate { private String[] names = new String[10]; private int index = 0; public void add(Object obj) { if (index < names.length) { names[index++] = (String) obj; } } public void remove(Object obj) { for (int i = 0; i < index; i++) { if (obj.equals(names[i])) { for (int j = i; j < index - 1; j++) { names[j] = names[j + 1]; } names[--index] = null; break; } } } public Iterator iterator() { return new ConcreteIterator(names); } } // 客户端 public class Client { public static void main(String[] args) { Aggregate aggregate = new ConcreteAggregate(); aggregate.add("张三"); aggregate.add("李四"); aggregate.add("王五"); Iterator iterator = aggregate.iterator(); while (iterator.hasNext()) { System.out.println(iterator.next()); } } }
在上面的示例中,抽象迭代器接口定义了访问和遍历聚合对象的方法,具体迭代器实现了该接口,并且用于具体实现访问和遍历聚合对象的操作。抽象聚合类接口定义了聚合对象的基本方法,具体聚合类实现了该接口,并且用于具体实现聚合对象的操作。文章源自新逸网络-https://www.xinac.net/9364.html
迭代器模式的优点是可以将访问和遍历聚合对象的操作与聚合对象本身分离开来,从而提高系统的灵活性和可扩展性。同时,由于迭代器模式提供了一种统一的访问接口,因此可以方便地切换不同的迭代器实现,例如顺序迭代、逆序迭代等。但是需要注意的是,由于迭代器模式需要额外的迭代器对象,因此可能会增加系统的复杂度和内存消耗。文章源自新逸网络-https://www.xinac.net/9364.html 文章源自新逸网络-https://www.xinac.net/9364.html
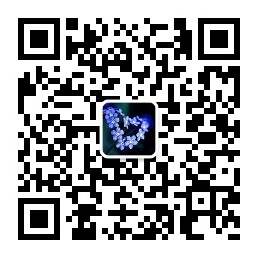
评论